https://github.com/google/iosched
https://github.com/google/iosched/blob/master/doc/BUILDING.md
https://github.com/google/iosched/blob/master/doc/CUSTOM.md
Wednesday, September 17, 2014
print server wireless issue
I have a CISCO router and some times my wireless connection to print server just get lost. I was using D-Link DPR-1260. Then I thought may be the print server is too old.
So I bought a Hawking HMPS2U and found out after I setup for wireless and everything worked fine. But when I power off, then I lost wireless connection.
So I called, the tech guy told me my Cisco router has to be configured to use channel 11, not to use Auto.
So I get to Cisco router and changed the connection to use channel 11, then power off/on both print server and both worked fine.
Oops, I wasted time and money but learned a lesson. My guess is just tuition. Let me see if I can resell this print server or ???
So I bought a Hawking HMPS2U and found out after I setup for wireless and everything worked fine. But when I power off, then I lost wireless connection.
So I called, the tech guy told me my Cisco router has to be configured to use channel 11, not to use Auto.
So I get to Cisco router and changed the connection to use channel 11, then power off/on both print server and both worked fine.
Oops, I wasted time and money but learned a lesson. My guess is just tuition. Let me see if I can resell this print server or ???
Saturday, September 13, 2014
Android SVG
http://stackoverflow.com/questions/25006511/how-do-i-render-an-svg-in-an-android-app
http://www.codeproject.com/Articles/130791/Android-ImageView-with-SVG-Support
http://stackoverflow.com/questions/18356098/having-issue-on-real-device-using-vector-image-in-android-svg-android
I managed to display an SVG using a
WebView
, since that is more than capable of rendering an SVG:webView.loadUrl("file:///android_res/drawable/file.svg"); // point it to the SVG
webView.setBackgroundColor(0x00000000); // set the background to transparent
This makes it render much like a transparent PNG would in an
ImageView
. The only caveat is that the SVGmust use the viewBox
attribute and not use the height
or width
attributes, to ensure it resizes properly.http://stackoverflow.com/questions/18356098/having-issue-on-real-device-using-vector-image-in-android-svg-android
use visual studio for android development
http://stackoverflow.com/questions/1371939/how-can-i-use-ms-visual-studio-for-android-development
image viewer
http://stackoverflow.com/questions/24416496/fragment-for-image-viewer-android
http://www.mkyong.com/android/android-imageview-example/
http://www.mkyong.com/android/android-imageview-example/
Thursday, September 11, 2014
Wednesday, September 10, 2014
Android asset folder
http://android-er.blogspot.com/2013/11/assets-folder-in-android-studio.html
assets folder in Android Studio
Android Studio is a new Android development environment based on IntelliJ IDEA, Gradle-based build support. It have different project structure.
In Eclipse, assets is in /assets folder under your project. In Android Studio, it is in /src/main/assets. In both Eclipse and Android Studio, /assets have the same level of /res folder.
Compare with the exercise of "Embed html using Google Maps JavaScript API v3 in Android App", where /assets/simplemap.html is a asset file of HTML. In Android Studio, it in /src/main/assets/simplemap.html. In both case, it can be accessed with "file:///android_asset/simplemap.html".
Uri path = Uri.parse("android.resource://com.androidbook.samplevideo/raw/myvideo");
In Eclipse, assets is in /assets folder under your project. In Android Studio, it is in /src/main/assets. In both Eclipse and Android Studio, /assets have the same level of /res folder.
Compare with the exercise of "Embed html using Google Maps JavaScript API v3 in Android App", where /assets/simplemap.html is a asset file of HTML. In Android Studio, it in /src/main/assets/simplemap.html. In both case, it can be accessed with "file:///android_asset/simplemap.html".
Note to everyone, you have to use AssetManager with this approach. If you're trying to use asset URIs to later replace with http:// in ImageView.setImageUri, that approach will not work (note: You should download images in a background thread, rather than using ImageView.setImageUri unless performance is not a concern). Otherwise, for Android 2.2 ImageView supports URIs but they have to be in android.resource form. So for images, you have to put them in the res/raw folder if you aren't going to use AssetManager directly and then the URL android.resource://[package name]/" + R.raw.id ... From http://androidbook.blogspot.com/2009/08/referring-to-android-resources-using.html :
"android.resource://[package]/[res type]/[res name]"Uri path = Uri.parse("android.resource://com.androidbook.samplevideo/raw/myvideo");
Tuesday, September 9, 2014
webview loading local html5
Working code below to load Chinese contents, a1.html is in src/main/assets
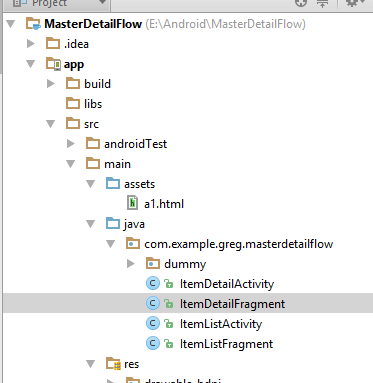
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.fragment_item_detail, container, false);
WebView myWebView = ((WebView) rootView.findViewById(R.id.website_detail));
// Show the dummy content as text in a TextView.
if (mItem != null) {
WebSettings settings = myWebView.getSettings();
settings.setDefaultTextEncodingName("utf-8");
settings.setSupportZoom(true);
settings.setBuiltInZoomControls(true);
//myWebView.loadUrl(mItem.website_url);
myWebView.loadUrl("file:///android_asset/a1.html");
// line below will set web page inside web view
myWebView.setWebViewClient(new WebViewClient());
}
return rootView;
}
Code listing at the bottom is the complete code include hiding the zoom control on the UI.
below is what I have researched links:
http://stackoverflow.com/questions/5125851/enable-disable-zoom-in-android-webview
http://stackoverflow.com/questions/18602676/android-studio-how-to-load-html5-content-from-a-local-path-using-webview
http://myexperiencewithandroid.blogspot.de/2011/09/android-loaddatawithbaseurl.html
http://blog.tourizo.com/2009/02/how-to-display-local-file-in-android.html
-------------------------------------------------------------
http://www.techjini.com/blog/2009/01/10/android-tip-1-contentprovider-accessing-local-file-system-from-webview-showing-image-in-webview-using-content/
I think if your HTML data can have a link like
<img src=content://com.tourizo.android.localfile/foo.jpg>
-------------------------------------------------------------
final String mimeType = "text/html";
final String encoding = "utf-8";
WebView wv;
try {
InputStream is = getAssets().open("test.html");
int size = is.available();
// Read the entire asset into a local byte buffer.
byte[] buffer = new byte[size];
is.read(buffer);
is.close();
// Convert the buffer into a string.
String strContent = new String(buffer);
wv = (WebView) findViewById(R.id.webview);
wv.loadData(strContent, mimeType, encoding);
} catch (IOException e) {
// Should never happen!
throw new RuntimeException(e);
}
}
-------------------------------------------------------------
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.fragment_item_detail, container, false);
WebView myWebView = ((WebView) rootView.findViewById(R.id.website_detail));
// Show the dummy content as text in a TextView.
if (mItem != null) {
WebSettings settings = myWebView.getSettings();
settings.setDefaultTextEncodingName("utf-8");
settings.setSupportZoom(true);
settings.setBuiltInZoomControls(true);
//myWebView.loadUrl(mItem.website_url);
myWebView.loadUrl("file:///android_asset/a1.html");
// line below will set web page inside web view
myWebView.setWebViewClient(new WebViewClient());
}
return rootView;
}
Code listing at the bottom is the complete code include hiding the zoom control on the UI.
below is what I have researched links:
http://stackoverflow.com/questions/5125851/enable-disable-zoom-in-android-webview
http://stackoverflow.com/questions/18602676/android-studio-how-to-load-html5-content-from-a-local-path-using-webview
http://myexperiencewithandroid.blogspot.de/2011/09/android-loaddatawithbaseurl.html
http://blog.tourizo.com/2009/02/how-to-display-local-file-in-android.html
-------------------------------------------------------------
http://www.techjini.com/blog/2009/01/10/android-tip-1-contentprovider-accessing-local-file-system-from-webview-showing-image-in-webview-using-content/
I think if your HTML data can have a link like
<img src=content://com.tourizo.android.localfile/foo.jpg>
-------------------------------------------------------------
final String mimeType = "text/html";
final String encoding = "utf-8";
WebView wv;
try {
InputStream is = getAssets().open("test.html");
int size = is.available();
// Read the entire asset into a local byte buffer.
byte[] buffer = new byte[size];
is.read(buffer);
is.close();
// Convert the buffer into a string.
String strContent = new String(buffer);
wv = (WebView) findViewById(R.id.webview);
wv.loadData(strContent, mimeType, encoding);
} catch (IOException e) {
// Should never happen!
throw new RuntimeException(e);
}
}
-------------------------------------------------------------
package com.example.greg.masterdetailflow;
import android.os.Bundle;
import android.app.Fragment;
import android.view.LayoutInflater;
import android.view.MotionEvent;
import android.view.View;
import android.view.ViewGroup;
import android.webkit.WebSettings;
import android.webkit.WebViewClient;
import android.widget.TextView;
import android.webkit.WebView;
import android.widget.ZoomButtonsController;
import com.example.greg.masterdetailflow.dummy.DummyContent;
/**
* A fragment representing a single Item detail screen.
* This fragment is either contained in a {@link ItemListActivity}
* in two-pane mode (on tablets) or a {@link ItemDetailActivity}
* on handsets.
*/
public class ItemDetailFragment extends Fragment {
ZoomButtonsController zoom_control = null;
/**
* The fragment argument representing the item ID that this fragment
* represents.
*/
public static final String ARG_ITEM_ID = "item_id";
/**
* The dummy content this fragment is presenting.
*/
private DummyContent.DummyItem mItem;
/**
* Mandatory empty constructor for the fragment manager to instantiate the
* fragment (e.g. upon screen orientation changes).
*/
public ItemDetailFragment() {
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
if (getArguments().containsKey(ARG_ITEM_ID)) {
// Load the dummy content specified by the fragment
// arguments. In a real-world scenario, use a Loader
// to load content from a content provider.
mItem = DummyContent.ITEM_MAP.get(getArguments().getString(ARG_ITEM_ID));
}
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.fragment_item_detail, container, false);
WebView myWebView = ((WebView) rootView.findViewById(R.id.website_detail));
// Show the dummy content as text in a TextView.
if (mItem != null) {
final WebSettings settings = myWebView.getSettings();
settings.setDefaultTextEncodingName("utf-8");
settings.setSupportZoom(true);
settings.setBuiltInZoomControls(true);
if (android.os.Build.VERSION.SDK_INT >= android.os.Build.VERSION_CODES.HONEYCOMB) {
// Use the API 11+ calls to disable the controls
settings.setBuiltInZoomControls(true);
settings.setDisplayZoomControls(false);
} else {
// Use the reflection magic to make it work on earlier APIs
getControls();
}
if (zoom_control!=null && zoom_control.getZoomControls()!=null)
{
// Hide the controlls AFTER they where made visible by the default implementation.
zoom_control.getZoomControls().setVisibility(View.GONE);
}
//myWebView.loadUrl(mItem.website_url);
myWebView.loadUrl("file:///android_asset/a1.html");
// line below will set web page inside web view
myWebView.setWebViewClient(new WebViewClient());
}
return rootView;
}
private void getControls() {
try {
Class webview = Class.forName("android.webkit.WebView");
//Method method = webview.getMethod("getZoomButtonsController");
zoom_control = (ZoomButtonsController) webview.getMethod("getZoomButtonsController").invoke(this, null);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Webview when click on URL link stays inside webview
import android.webkit.WebView;(Alt-enter to add this)
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.fragment_item_detail, container, false);
WebView myWebView = ((WebView) rootView.findViewById(R.id.website_detail));
// Show the dummy content as text in a TextView.
if (mItem != null) {
myWebView.loadUrl(mItem.website_url);
myWebView.setWebViewClient(new WebViewClient());
}
return rootView;
}
http://www.techotopia.com/index.php/An_Android_Studio_Master/Detail_Flow_Tutorial
http://developer.android.com/guide/webapps/webview.html#HandlingNavigation
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.fragment_item_detail, container, false);
WebView myWebView = ((WebView) rootView.findViewById(R.id.website_detail));
// Show the dummy content as text in a TextView.
if (mItem != null) {
myWebView.loadUrl(mItem.website_url);
myWebView.setWebViewClient(new WebViewClient());
}
return rootView;
}
http://www.techotopia.com/index.php/An_Android_Studio_Master/Detail_Flow_Tutorial
http://developer.android.com/guide/webapps/webview.html#HandlingNavigation
Monday, September 8, 2014
Master detail flow
http://www.techotopia.com/index.php/An_Android_Studio_Master/Detail_Flow_Tutorial
Sunday, September 7, 2014
Friday, September 5, 2014
Android turn from portrait mode to landscape mode
It will destroy the current activity and recreate activity all 6 life cycle methods
onCreate
onStart
onRsume
onPause
onStop
onDestroy
onCreateOptionMenu(Menu:menu)
Android starting point
Android. Intent. Action. Main
Android. Intent. Category. LAUNCHER
Indicates icon appear at apps screen.
Subscribe to:
Posts (Atom)